C-programming-project
Hi i have a C language programming project for my computer science class that I am having difficulty with. This is a continuation of my previous code that I made so I will provide the code I have and what needs to be done is add another option into the code. I will attach a sample execution where when the user picks number 4 so they can play a game of bingo. The IDE I currently use is Dev C++. If there are any questions or anything confusing please let me know as I need this code done asap! thank you
For this project this is what my instructor told us.
Create a program that will:
- do everything from program 2 (create bingo cards), if you didn’t get this working just right go see TAs/professor As Soon As Possible
- Add an option 4 to your menu for Play Bingo
- read in a bingo call (e,g, B6, I17, G57, G65)
- checks to see if the bingo call read in is valid (i.e., G65 is not valid)
- marks all the boards that have the bingo call
- checks to see if there is a winner, for our purposes winning means
- 5 tokens in a row or column
- 5 tokens in a diagonal
- 1 token in each of the 4 corners of the game board
- Loop accepting bingo calls until there is a winner, and display an appropriate message specifying which user won, print their bingo card, and how they won (5 in a row with calls B6, I20, …; 5 in a column with calls …; 5 on a diagonal with calls … ; 4 corners with calls …)
Grading:
35 points style per @10 (We will be looking for functions now. So having a function to check for a winner, or initialize the board or, ….)
20 points checking for winner code, we expect to see multiple functions here, 1 function that checks for each way of winning (row, col, diag, 4 corners)
10 points checks for valid bingo calls
10 points appropriate message for winner displayed
10 points game play works until winner is found
5 points recording which calls were received,
10 points marking the boards with the calls that have been received
This is my current code right now.
—————————————————————————–
#include <stdio.h>
#include <stdlib.h>
int getRandomNumber(int max, int min)
{
return min + (rand() % (max – min + 1));
}
int maxCol(int Col)
{
return ((Col + 1) * 15);
}
int minCol(int Col)
{
return ((Col * 15) + 1);
}
void bingoCard(int cards[5][10][5][5], int Players, int Cards)
{
int i; // i and j are used for the for loops
int j;
printf(” B I N G On”);
for(i = 0; i < 5; i++)
{
for(j = 0; j < 5; j++)
{
if(j == 2 && i == 2)
{
printf(” free “);
}
else
{
printf(” %2d “, cards[Players][Cards][i][j]);
}
}
printf(“n”);
}
printf(“n”);
}
int main()
{
srand(time(NULL));
int numofPlayers;
int numofCards;
int number;
int i;
int j;
int k;
int l;
int m;
int numofRows = 5;
int numCols = 5;
int bingoCards[5][10][5][5];
int count[75];
int Var = 0;
int Generator;
int pick = 1;
printf(“Enter number of players: “);
scanf(“%d”, &numofPlayers);
if(numofPlayers > 5)
{
printf(“Max players allowed are 5, please enter a number less than 5n”);
printf(“Enter number of players: “);
scanf(“%d”, &numofPlayers);
}
printf(“Enter the number of BINGO cards per player: “);
scanf(“%d”, &numofCards);
if(numofCards > 10)
{
printf(“Max number of cards are 10, please enter a number less than 10n”);
printf(“Enter the number of BINGO cards per player: “);
scanf(“%d”, &numofCards);
}
printf(“You are playing a game with %d players and each player will have %d cardsn”, numofPlayers, numofCards);
printf(“We have generated %d bingo cards.n”, (numofPlayers * numofCards)); // This will do the math for how many people are playing and how many cards will be used.
for(i = 0; i < numofPlayers; i++)
for(j = 0; j < numofCards; j++)
{
for(k = 0; k < numCols; k++)
{
for(l = 0; l < numofRows; l++)
{
while(pick)
{
number = getRandomNumber(minCol(k), maxCol(k));
Var = 0;
for(m = 0; m < l; ++m)
{
if(bingoCards[i][j][m][k] == number)
{
Var = 1;
}
}
if(Var == 0)
{
break;
}
}
bingoCards[i][j][l][k] = number;
}
}
}
}
for(i = 0; i < 75; ++i)
{
count[i] = 0;
}
for(i = 0; i < numofPlayers; i++)
{
for(j = 0; j < numofCards; j++)
{
for(k = 0; k < numofRows; k++)
{
for(l = 0; l < numCols; l++)
{
if(!(k == 2 && l == 2))
{
count[bingoCards[i][j][k][l] – 1]++;
}
}
}
}
}
while(pick)
{
printf(“Please choose an option from the following menu:n”);
printf(“1) Display a bingo cardn”);
printf(“2) Run a histogram across all bingo cards generatedn”); //Display menu options for the user
printf(“3) Exitn”);
printf(“You have chosen “);
scanf(“%d”, &i);
switch(i)
{
case 1:
printf(“Enter the player and players card you would like to display,n”);
printf(“First player is 1, last player is %dn”, numofPlayers);
printf(“First card is 1, last card is %dn”, numofCards);
scanf(“%d %d”, &j, &k);
bingoCard(bingoCards, (j – 1), (k – 1));
break;
case 2:
for(j = 0; j < 75; ++j)
{
Generator = (rand() % 25) + 1;
if(j < 10)
{
printf(“%d”, j + 1);
}
else
{
printf(“%d”, j + 1);
}
if(j < 10 && Generator < 10)
{
printf(“-%d “, Generator);
}
else if(j < 10 && Generator >= 10)
{
printf(“-%d “, Generator);
}
else
{
printf(“-%d “, Generator);
}
if(j % 10 == 9)
{
printf(“n”);
}
}
printf(“n”);
break;
case 3:
printf(“GoodBye”);
return 0;
default:
printf(“Invalid choice, try again.n”);
break;
}
}
return 0;
Do you need a similar assignment done for you from scratch? We have qualified writers to help you. We assure you an A+ quality paper that is free from plagiarism. Order now for an Amazing Discount!
Use Discount Code "Newclient" for a 15% Discount!
NB: We do not resell papers. Upon ordering, we do an original paper exclusively for you.
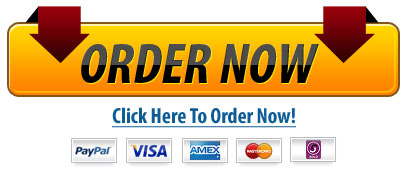